There has always been a great chasm between artists of any media and the nerds that work to support them. There are many reasons for this. While not always the case, programmers generally have spent time in university learning math and algorithm design. Artists generally have spent the same time smoking pot and throwing feces at a canvas. I'm a self taught programmer that went to a commercial school for audio. I smoked a lot of pot but also spent time learning the maths and watching Bob Ross paint on hangover Sunday.
This lesson is about how the nerds should approach the hippies so we can all get along.
The chasm is real, it is called "Toolset Chasm the Precipice of Sorrow" it was caused by years of book learning, passion, and 2 tribes that speak totally different languages.
We can break it down to wants and needs. Art needs tools, art wants the most transparent tools that allow them to produce beautiful things that make us laugh and cry. Programmers need tasks, programmers want to deliver the most elegant solutions that they can deliver in the shortest amount of time so they can move on to other tasks. They are equally passionate but the love is a different kind.
In the many job interviews I have had I describe myself as a person that can translate the unreasonable demands of the artists into reasonable tasks for the engineers. I'm really good at it "pats self on back". It is a rare skill.
Here is how programmers can join club awesome and roll like I
1. There is no such thing as programmer art. Programmer art is a text box, a spread sheet, a text file or some human (read super nerd) readable schema that represent the "content"
2. There is no such thing as content. It is art. Someone spent hours with some fancy gadgets producing the equivalent of their soul in digital form. Respect that, they aren't retards because they can't decompose a rotation matrix though they could be reards if they require IT every time they want to print said digital soul.
3. Stop saying No all of the time. Any tool is nothing more than a glorified text editor, they are not hard to make. There are still cases where No is still the appropriate response. Just not all of the time.
4. You have time. If an artist ask for a tool that uses a water gun to produce the assets for the game then go get a super soaker and build that shit. It is cool. The artists will make you a bacon apple pie or draw you your next space invader tattoo. What is sufficient is almost never what is most productive. Stop running to "There isn't enough time in the schedule". An unproductive artists will have you sitting updating facebook status until you need a catheter.
5. Its usually worth the risk. The other mantra of the programmer " At this point its too risky". See point 4.
6. Artists are shit typists. They don't want a text box that takes 3 floats to represent a position in space, they want an "Over there" orb of awesome.
Box of FAIL
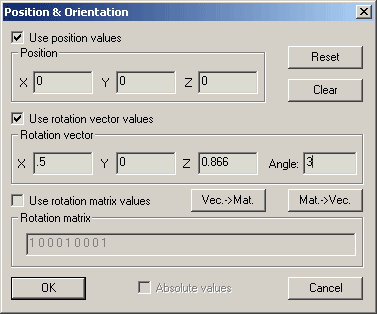
ORB of AWESOME
7. A lot of the work is done for you already. Look at the creation tools the artists like to use. Most of them were bult by artists that are shit programmers. Steal their cool stuff and make it work better. Faders, color pickers, spline controls, waveform editors. There is a bounty of very nice controls that will have bacon on your plate in no time.
There are more rules but you are smart enough to figure them out. Start with making it beautiful, then make it work. I kmow its contrary to your very being but its what art needs.
Here's how artists can join club awesome and live with the hot ones:
1. Stop whining. The big bad programmer is not there to crush your dreams, he's there to build the machine that will get your life's work into the hands of the sweaty masses. Programmers are just as sensitive and reactive as you are, just for different reasons. Try not punching them in the face or writing 37 page emails about how the lack of an orb of awesome will completely halt your ability to work.
2. Teach the programmer. They are smart folks at a level equal to your talents. Show them how you do your stuff. They will not put you out of a job but they will have a better idea of the tools you really need to make the beautiful.
3. Learn from the programmer. Don't learn C++. You'll check in dangling pointers and break the build (Something I have done, twice). Learn a bit about how your stuff gets turned in to their stuff. Your talent is at a level equal to their smarts.
4. There are people that can do both. Usually they are called tech artists. If you don't have one, hire one today. They are thoroughly good. They are like tour guides, they know all of the cool spots in town and can translate with the locals.
5. You do not have time. Well you do just not unlimited time. Understand the schedule and build it with the programmers. Instead of needing 3 months to produce 10 different 5 meg cat ass textures. Build one good one and take it to a programmer, they can make something procedural that can reuse one cat ass texture to look like 10.
6. You are broke. You have a budget that is never enough to get all of the stuff you want. Welcome to the life of an artist. If you have to have all of those cat asses, see point 5.
7. Buy them beer. Programmers like beer. Share the beer with them. After a few pints they will be pulling out the pocket protector and will listen to your craziest ideas. Some of them may even make it past hangover Sunday and get in to the game.
Following these guidelines will begin to fill the chasm with love. A game team is a complete team of art and science. When there is harmony you will begin to see beautiful things take place. The nerds will talk to you. It will be like an episode of glee, but without the Journey cover tunes.
Both tribes are free to disagree with me. I accept insult in either language.
C. Artist, Programmer, Chasm of Sorrow filler.